티스토리 뷰
왕초보를 위한 iOS 앱개발 - 야곰닷넷
코딩의 '코'자를 몰라도 시작해 볼 수 있는 iOS 앱만들기! 왕초보를 위한 iOS 앱개발 입문편입니다.
yagom.net
1. Xcode 프로젝트 만들기
To-Do List
-
Add Slider
-
Add 'HIT Button'
-
Receive value changed events from the slider
-
Add 'RESET Button'
-
Add labels presentin information
-
Generate the random number
-
Compare the random number with input number
-
Show alerts
-
Implement 'reset' feature
-
Add 'Credit' view
2. UI와 이벤트
2-1. UIKit(User Interface Kit)과 스토리보드
- 라이브러리 단축키: cmd + shift + L
2-2. 스토리보드에 UI 구성요소 얹기
- Simulator 배율 단축키: cmd + 숫자
- 구성요소 삭제: Delete
- 변경하면 정지 후 다시 실행
2-3. 슬라이더와 HIT 버튼 추가하기
- Attribute inspector : 화면 요소들의 속성들을 설정해줄 수 있다.
- 오류
- Attribute inspector에서 지정한 값이 시뮬레이터 상에서 1로 나옵니다. 그냥 화면에 나오는 대로 따라하고 엔터도 치고 스토리보드 상에서 0 - 30의 가운데인 15 위치에 슬라이더가 있는 걸 확인했는데 실행만하면 1에 위치해서 나옵니다.
2-4. 슬라이더 값의 변경 파악하기
-
코드를 작성할 때는 대소문자와 띄어쓰기가 정말 중요하다
-
Slider에 대한 값 표시하는 Action 만들기
- ViewController.swift
- @IBAction func sliderValueChanged() 추가
class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view. } @IBAction func sliderValueChanged(_ sender: UISlider) { print(sender.value) } }
-
Main.storyboard.swift
-
View Controller 클릭
-
Connection Inspector → Received Actions 클릭
-
Slider 연결 → Value Changed 클릭
-
slider를 움직일 때마다 값이 표시된다.
-
- ViewController.swift
2-5. Action과 Outlet
-
슬라이더와 버튼을 연결하여 버튼을 클릭하면 값 확인하기
- ViewController.swift
- @IBOutlet, @IBAction func touchUpHitButton() 추가
class ViewController: UIViewController { @IBOutlet weak var slider: UISlider! override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view. } @IBAction func sliderValueChanged(_ sender: UISlider) { print(sender.value) } @IBAction func touchUpHitButton(_ sender: UIButton) { print(slider.value) } }
-
Main.storyboard
-
View Controller 클릭
-
Connection Inspector → Outlets > slider → slider로 연결
-
Connection Inspector → Referencing Action > touchUpHitButton 클릭 → button과 연결
-
버튼을 클릭하면 값이 표시된다.
-
- ViewController.swift
2-6. 이벤트 기반 프로그래밍(Event Driven Programming)
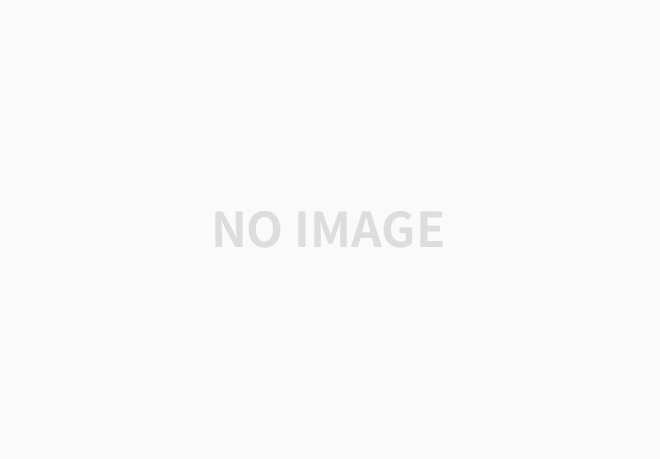
Event Driven Programming
- 이벤트를 수신하는 역할
- 사용자가 이 버튼을 탭하게 되면 이러한 코드를 실행해야지
- push notification이 오면 이런 걸 실행해야지
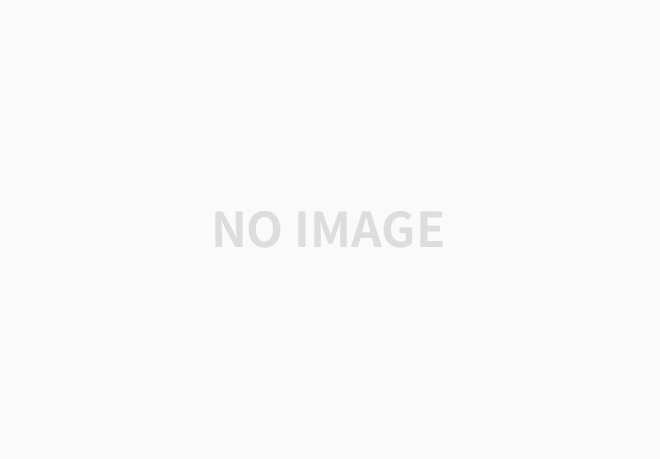
- 사용자의 이벤트가 없는 경우라면 코드는 실행되지 않고 무한정 기다리게 된다.
2-7. [도전!] 초기화 버튼 만들기
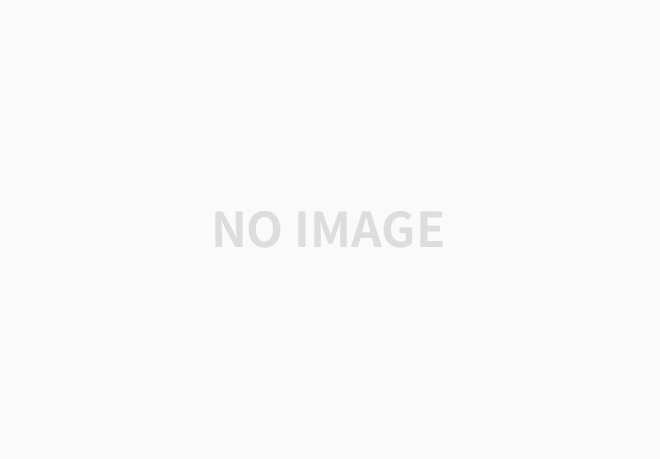
- ViewController.swift
-
@IBOutlet, @IBAction func touchUpHitButton() 추가
-
모든 연결 이후 함수 이름을 변경하고자 한다면 오른쪽 클릭으로 refactor로 바꿔야 한다!
import UIKit class ViewController: UIViewController { @IBOutlet weak var slider: UISlider! override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view. } @IBAction func touchUpResetButton( _sender: UIButton) { print("touch up reset button") } }
- Main.storyboard
-
View Controller 클릭
-
Connection Inspector → Referencing Action > touchUpResetButton 클릭 → RESET 버튼과 연결
-
앱 동작화면

2-8. 레슨2 정리
Lesson 2 Wrapping Up
- UIkit & Storyboard
- Adding UI components on the storyboard
- Adding Slider and HIT Button
- Receiving value changed event from slider
- Getting current value of slider
- Event driven programming
- Challenge - Adding RESET Button
To-Do List
-
Add Slider
-
Add 'HIT Button'
-
Receive value changed events from the slider
-
Add 'RESET Button'
-
Add labels presentin information
-
Generate the random number
-
Compare the random number with input number
-
Show alerts
-
Implement 'reset' feature
-
Add 'Credit' view
Quiz
Q2) 사용자 인터페이스와 사용자 경험을 위한 대부분의 구현체를 포함하는 프레임워크의 이름은 무엇일까요?
Foundation
Swift
Core UI
UIKit
Core Foundation
Q) 클래스의 액션 메서드와 인터페이스 빌더의 이벤트를 연결하기 위해 메서드 앞에 붙이는
어노테이션(Annotation)과 클래스의 프로퍼티와 인터페이스 빌더의 요소를 연결하기 위해
프로퍼티 앞에 붙이는 어노테이션(Annotation)을 빈칸에 알맞게 채워보세요.
@___ / @___
Q) UIKit의 요소를 크게 입력과 출력으로 구분한다고 할 때, 다음 중 다른 한 가지는 무엇일까요?
Views and Controls
View Controllers
Animation and Haptics
Windows and Screens
Touches, Presses, and Gestures
Q) Xcode에서 앱에 사용될 리소스(이미지, 음악 파일 등)의 집합을 나타내는 요소는 무엇일까요?
Inspector
Attribute
Asset
Files
Target
Q) iOS 앱에서는 여러 [ ]에 의해 실행할 코드가 결정되는데요.
이것을 ‘[ ] 기반 프로그래밍’ 혹은 ‘[ ] 주도 프로그래밍’이라고 표현합니다.
[ ]는 사용자 혹은 시스템 등에서 여러 상황에 전달합니다. 빈 칸에 알맞은 표현은 무엇일까요?
함수
이벤트
클래스
객체
액션
'iOS' 카테고리의 다른 글
오토레이아웃 정복하기#1 (0) | 2021.04.01 |
---|---|
[iOS] 왕초보를 위한 iOS 앱개발 6 (0) | 2021.03.15 |
[iOS] 왕초보를 위한 iOS 앱개발 5 (0) | 2021.03.12 |
[iOS] 왕초보를 위한 iOS 앱개발 4 (0) | 2021.03.12 |
[iOS] 왕초보를 위한 iOS 앱개발 3 (0) | 2021.03.12 |
- Total
- Today
- Yesterday
- 흐름제어구문
- 훈련법
- 범위연산자
- playground
- conditional
- 객체지향 생활체조
- 삼항연산자
- 옵셔널
- overflow
- variables
- scope
- 구문이름표
- optional
- swift
- 오버플로우연산자
- 반복문
- enumerations
- 결합성
- 비트연산자
- continue
- Constants
- 오토레이아웃
- 전산구문 기초용어
- labeled
- datatypes
- Functions
- 산술연산자
- 세자리수마다 콤마넣기
- RawValues
- iOS View Life Cycle
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |